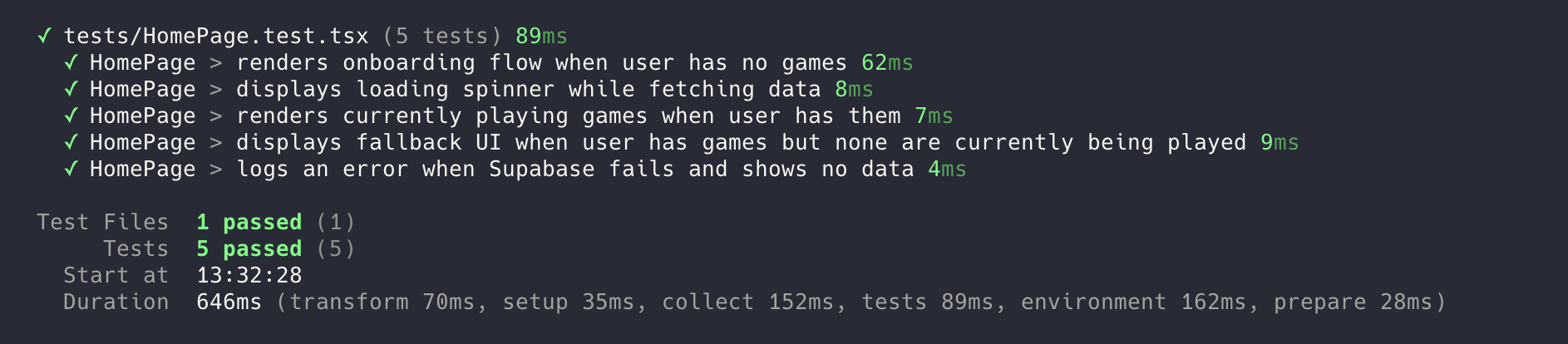
Lets get technical... and hormonal?!
I’ve been trying to continue building the AI features for Backlog Explorer this week, but honestly? I’ve been distracted.
Not in the “I’m procrastinating” kind of way… more like… my brain just didn’t want to do new things. It wanted to go deep instead. Why? Because, hi, I’m a woman with a menstrual cycle. And that means I move through different phases each month — creative, energetic phases, and more introspective, inward ones.
Right now, I’m in my inner autumn (aka luteal phase), and I always feel a bit slower, more analytical, and way more likely to deep-dive into something I want to fix.
So today, when I felt that inner pull to go deep instead of build, I remembered Martin Fowler’s Refactoring book sitting on my desk — and thought, “Okay. Let’s actually try this.”
I opened up my HomePage.tsx
file and said:
“You know what? I’m gonna refactor this mess.”
Intimidated by refactoring — but ready to try
The component was a tangled web — a giant useEffect
trying to juggle too many tasks at once.
It fetched the user, set state, checked onboarding, fetched games, formatted them, managed loading logic… all bundled into one block. I wrote the code, then never looked back because, well, it worked (yay!). But reading it afterward? Total gibberish.
I was intimidated by the idea of refactoring it. What if I broke something? What if I made it worse? The code was messy, sure, but it was working. That felt like enough — or at least, that’s what I told myself.
Click to view the original useEffect if you're into the technical stuff
useEffect(() => {
const fetchCurrentGames = async () => {
try {
const {
data: { user },
} = await supabase.auth.getUser()
if (!user) {
navigate('/login')
return
}
const firstName = user.user_metadata?.full_name?.split(' ')[0] || ''
setUserName(firstName)
const { count: totalGames } = await supabase
.from('user_games')
.select('*', { count: 'exact', head: true })
.eq('user_id', user.id)
setShowOnboarding(totalGames === 0)
const { data: games, error } = await supabase
.from('currently_playing_with_latest_note')
.select('*')
.eq('user_id', user.id)
.order('note_created_at', { ascending: false, nullsFirst: false })
if (error) throw error
const formattedGames =
games?.map((g) => ({
id: g.game_id,
title: g.title,
image: g.image || g.background_image,
progress: g.progress,
platforms: g.platforms || [],
genres: [],
status: g.status,
nextIntent: g.next_session_plan?.intent || '',
nextNote: g.next_session_plan?.note || '',
})) || []
setCurrentGames(formattedGames)
} catch (error) {
console.error('Error fetching current games:', error)
} finally {
setLoading(false)
}
}
fetchCurrentGames()
}, [navigate])
Technically, it functioned. But it felt fragile, like it could break with the slightest touch. I was hesitant to change it because I didn’t want to risk breaking what was already working.
Honestly, my code wasn’t pretty - it was like a cluttered closet (don’t open the door!). But it got the job done.
Testing — a mystery until I tried it
I remembered how in bootcamp we learned testing, TDD, and writing clean code—and I was like:
“YES, I’m going to test all the things and build things the right way!!”
But… I never really got it.
I didn’t understand what tests were for, beyond just checking if the code worked.
I didn’t feel confident writing them.
And when I started working on Backlog Explorer, I skipped them completely.
I told myself I’d go back and add them later.
Besides, I thought, Why do I need tests? I can just open the app and visually check if it works.
So I never wrote them.
Leaning into menstrual cycle awareness — inner autumn focus
This time, I wrote tests before refactoring.
Why? Because Martin Fowler told me to. So I did. I trusted the book. 🙃
I wrote just a few:
- one for the onboarding state
- one for the loading spinner
- one for rendering currently playing games
- one for handling errors
Nothing fancy.
And suddenly… it felt like the beast got a makeover.
If I messed something up? The tests would catch it.
If I renamed something wrong? The tests would tell me.
If I broke onboarding logic? The tests would scream at me.
It was honestly wild. I finally understood. Like — ohhh, this is why people love tests. This is why TDD exists. This is why you don’t just visually check everything every time like a dummy.
It’s so cool.
I love seeing all the green check marks.
It’s actually… fun??
I’m enjoying refactoring. A lot.
The “great big scary” refactor — turning messy into maintainable
I created a helper file:
/src/pages/helpers/homePageHelpers.ts
And started pulling logic out into focused little functions:
getUserOrRedirect(navigate)
extractFirstName(user)
isNewUser(userId)
fetchFormattedCurrentGames(userId)
Each one does one thing.
Each one reads clearly.
Each one makes the useEffect
feel tameable.
Any fool can write code that a computer can understand. Good programmers write code that humans can understand. – Martin Fowler
The Result
Now my HomePage’s useEffect
looks like this:
useEffect(() => {
const loadUserHomepage = async () => {
try {
const user = await getUserOrRedirect(navigate)
if (!user) return
setUserName(extractFirstName(user))
setShowOnboarding(await isNewUser(user.id))
setCurrentGames(await fetchFormattedCurrentGames(user.id))
} catch (error) {
console.error('Error fetching current games:', error)
} finally {
setLoading(false)
}
}
loadUserHomepage()
}, [navigate])
As Martin Fowler describes in the very first chapter of the book, “…with good names, I don’t have to read the body of the function to see what it does.”
It breathes.
It flows.
This is the kind of code I want to come back to.
Is this real refactoring? Yes, it absolutely is.
I had a moment where I wondered, Is this really refactoring? It felt small, not flashy, not a big rewrite or a shiny new feature. But that’s the thing — refactoring isn’t about grand gestures. It’s about thoughtful, incremental improvements that make the code clearer and easier to work with.
This process reminded me that refactoring is a form of care — care for the code, and care for my future self (and anyone else who might read this code later).
It’s not just technical cleanup. It’s about reducing friction. Creating ease. Making space. And honestly, that mirrors what I’m learning to do for myself during my inner autumn, too — to reduce friction, slow down, and tend to what needs support. There’s something beautifully aligned about doing that in my code at the same time I’m doing it in my body.
Refactoring + Inner Autumn = Perfect Combo
This kind of work felt so aligned with my cycle.
It wasn’t fast or flashy.
It was quiet. Focused. Intentional.
I wasn’t building something new — I was tending to something that already existed. Making it stronger. Clearer. Easier to understand and easier to change or update in the future.
It also just… fit the kind of energy I had this week. I didn’t want to rush. I wanted to investigate deeply. I wanted to problem-solve meaningfully. And I wanted to make something that already existed feel better to work with—not just for me, but for future me too.
🧠 What I Learned
- Refactoring is creative too — just in a quieter, more grounded way
- Testing makes me feel more confident
- My cycle isn’t a blocker
- Recognizing when and how to improve messy but working code is a huge part of becoming a better developer
✨ Final Thought
Progress doesn’t always look like shipping new features or building something big.
Sometimes, it’s cleaning up what you’ve already made.
Sometimes, it’s learning to enjoy the parts that used to scare you.
And sometimes — especially for those of us with menstrual cycles — it’s about trusting the natural rhythms of our energy.
There are parts of the month when I feel wildly creative and inspired, and parts like now when I feel slower, more inward, more focused on fixing things. And that’s not a flaw — it’s a feature.
This week reminded me that I don’t have to fight my cycle. I can work with it.
And honestly? My code — and my nervous system — are better for it.